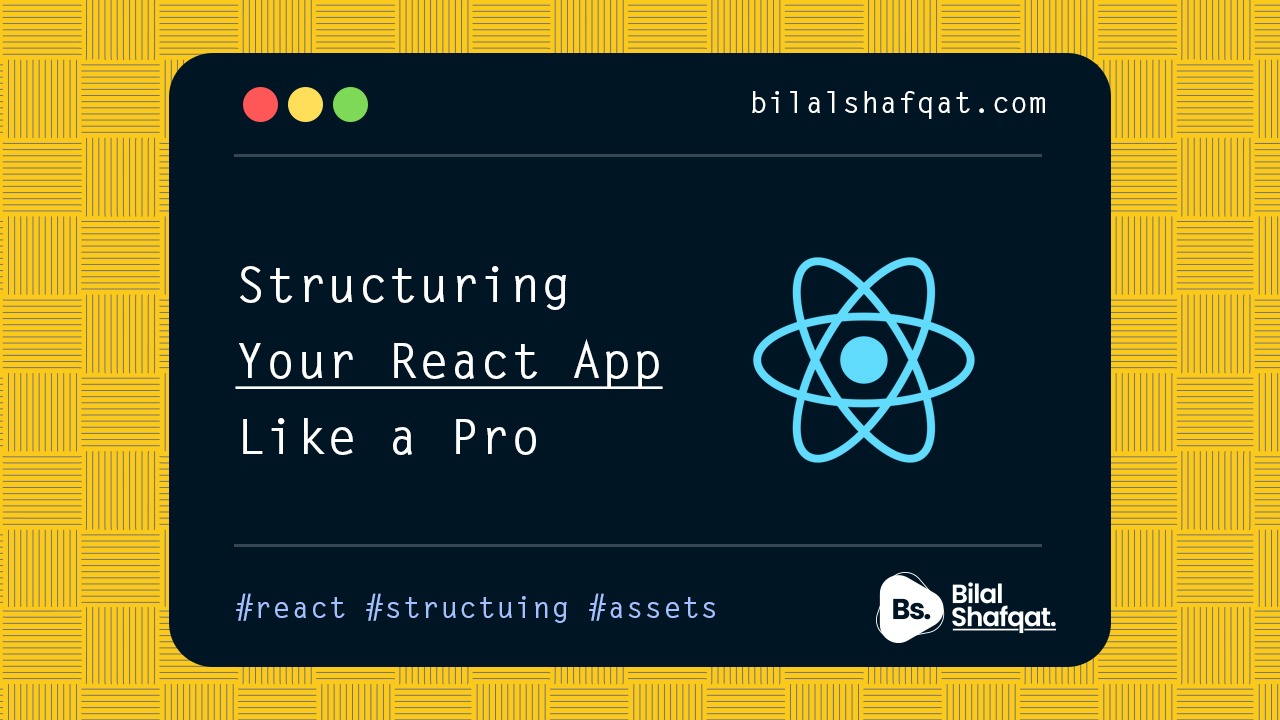
Structuring Your React App Like a Pro
- bilalshafqat42
- February 18, 2025
- Blog, React Js
- 1 Comment
Introduction: Why App Structure Matters
When developing a React application, maintaining a well-organized project structure is crucial for scalability, maintainability, and collaboration. A well-structured React app allows developers to navigate the codebase efficiently, scale features seamlessly, and debug issues with ease.
A poor project structure leads to messy code, duplicated logic, and unmanageable complexity as the app grows. By following a professional structure, you ensure your React project remains robust and future-proof.
This guide will help you structure your React application effectively by covering the following essential directories:
- Assets: Managing static files
- Styles: Organizing stylesheets and theme configurations
- Components: Building reusable UI elements
- Helpers: Creating utility functions for common operations
- Hooks: Custom React hooks for managing state and side effects
- Contexts: Centralized state management using React Context API
- Layouts: Structuring pages efficiently
Let’s dive into each section in detail.
Structuring Your React App
1. Assets – Managing Static Files
The assets directory contains static files such as images, fonts, icons, and other non-code resources used in the app. Keeping all assets in a dedicated folder ensures a clean and predictable project structure.
Best Practices:
- Store images in
/assets/images
and organize them by feature or category. - Keep icons in
/assets/icons
for better reusability. - Fonts should reside in
/assets/fonts
to maintain consistency across the app. - Avoid importing assets directly into components. Instead, use dynamic imports for better performance.
Example Directory Structure:
/assets ├── images │ ├── logo.png │ ├── banner.jpg │ └── user-avatar.png ├── icons │ ├── home.svg │ ├── search.svg └── fonts ├── OpenSans.ttf └── Roboto.ttf
2. Styles – Organizing Stylesheets and Themes
The styles directory is dedicated to global and component-specific stylesheets. Using a proper styling strategy enhances maintainability and ensures consistency in the app’s design.
Best Practices:
- Use SCSS or CSS Modules for modular styling.
- Maintain a global stylesheet (e.g.,
global.scss
orglobal.css
) for universal styles. - Store reusable variables, mixins, and theme settings separately.
- Consider using styled-components or Tailwind CSS for modern styling approaches.
Example Directory Structure:
/styles ├── global.scss ├── variables.scss ├── mixins.scss ├── themes │ ├── dark.scss │ ├── light.scss
3. Components – Reusable UI Elements
The components directory houses all reusable UI elements such as buttons, input fields, cards, and modals. Components should be modular, reusable, and follow the Single Responsibility Principle.
Best Practices:
- Use separate folders for each component.
- Keep related files (JSX, CSS, test files) within the same component folder.
- Maintain an index.js for easy imports.
- Categorize components into
UI
,Common
, andFeature-Specific
components.
Example Directory Structure
/components ├── Button │ ├── Button.jsx │ ├── Button.module.scss │ └── index.js ├── Modal │ ├── Modal.jsx │ ├── Modal.module.scss │ └── index.js
4. Helpers – Utility Functions
The helpers directory contains utility functions that simplify code reusability. This includes data formatting, API requests, and general-purpose functions.
Best Practices:
- Group related helper functions into separate files.
- Keep functions pure and reusable.
- Document each function for better understanding.
Example Directory Structure:
/helpers ├── formatDate.js ├── validateInput.js ├── apiRequests.js
5. Hooks – Custom React Hooks
The hooks directory stores reusable custom hooks to manage state and side effects efficiently.
Best Practices:
- Prefix hook names with
use
(e.g.,useAuth
,useFetch
). - Keep hooks focused on a single responsibility.
- Document how each hook should be used.
Example Directory Structure:
/hooks ├── useAuth.js ├── useFetch.js ├── useLocalStorage.js
6. Contexts – State Management with React Context API
The contexts directory holds context providers to manage global state across the app.
Best Practices:
- Separate concerns by creating multiple contexts (e.g., AuthContext, ThemeContext).
- Use React’s
useReducer
for complex state management. - Provide default values and clear documentation.
Example Directory Structure:
/contexts ├── AuthContext.js ├── ThemeContext.js ├── AppContext.js
7. Layouts – Structuring Pages
The layouts directory helps in defining page layouts such as headers, footers, and sidebars.
Best Practices:
- Create different layouts for different sections (e.g., AuthLayout, DashboardLayout).
- Keep layouts modular and reusable.
- Wrap pages with the appropriate layout component.
Example Directory Structure:
/layouts ├── MainLayout.js ├── DashboardLayout.js ├── AuthLayout.js
Frequently Asked Questions (FAQ)
1. Why is a structured React project important?
A structured project improves maintainability, makes debugging easier, and ensures scalability as your app grows.
2. Should I use separate folders for each component?
Yes, keeping each component in its own folder along with its styles and tests improves organization.
3. How do I decide when to use Context API?
Use Context API when state needs to be shared across multiple unrelated components, reducing prop drilling.
4. Can I combine multiple stylesheets into one?
Yes, but using modular stylesheets (CSS Modules, SCSS, Tailwind) helps maintain better structure and prevents conflicts.
5. Are hooks necessary for every project?
Not always, but they provide better state management and code reusability in functional components.
5 Must-Know React Component Patterns for Scalable Code - Bilal Shafqat
[…] long-term projects, it’s easy to end up with messy, repetitive, or hard-to-maintain code. But React gives us patterns — simple structures and ideas — that make our code easier to read, reuse, and […]