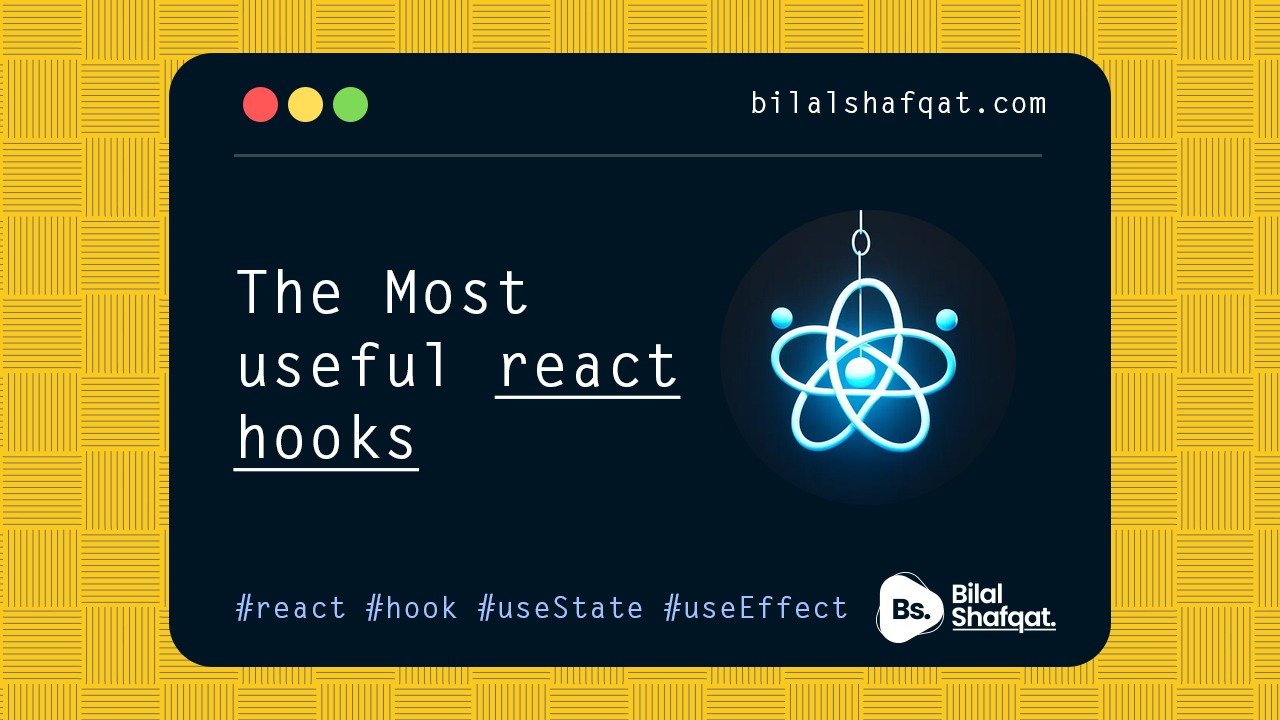
Most Useful React Hooks
- bilalshafqat42
- January 27, 2025
- Blog, React Js
- 0 Comments
Most Useful React Hooks
Discover the most essential React Hooks that every developer should know! From managing state with useState
to harnessing advanced features like useEffect
, useContext
, and more, this guide covers the top React Hooks with practical examples. Perfect for beginners and seasoned React developers, learn how to boost your productivity and write cleaner, more efficient code. Dive in to explore the power of React Hooks and take your frontend development skills to the next level!
1. useState() react hook
useState
is a React Hook that allows you to add state to functional components. It enables you to manage dynamic values within your component, such as user inputs, toggles, counters, or any data that may change over time.
const [state, setState] = useState(initialValue);
Parameters:
initialValue
: The initial state value. It can be any data type (e.g., string, number, object, array, etc.).state
: The current state value.setState
: A function used to update the state value.
Example:
1. Counter Example:
import React, { useState } from "react"; function Counter() { const [count, setCount] = useState(0); // Initial state is 0 return ( <div> <p>Current Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> <button onClick={() => setCount(count - 1)}>Decrement</button> </div> ); } export default Counter;
2. Input Example:
import React, { useState } from "react"; function InputForm() { const [name, setName] = useState(""); return ( <div> <input type="text" value={name} onChange={(e) => setName(e.target.value)} placeholder="Enter your name" /> <p>Your Name: {name}</p> </div> ); } export default InputForm;
Key Points:
- Re-renders: Updating the state via
setState
triggers a re-render of the component. - State is isolated: Each
useState
call creates its own state, even if used multiple times in the same component. - Lazy Initialization: If
initialValue
requires computation, you can pass a function touseState
:
const [value, setValue] = useState(() => expensiveComputation());
2. useEffect() react hook
useEffect
is a React Hook that lets you perform side effects in functional components. It’s a replacement for lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
in class components.
It runs after the render is committed to the DOM and can be used for a variety of purposes such as fetching data, subscribing to events, or manipulating the DOM.
useEffect(() => { // Side effect logic return () => { // Cleanup logic (optional) }; }, [dependencies]);
Parameters:
- Effect Function (
() => {}
): Contains the side-effect logic. - Dependency Array (
[dependencies]
): Determines when the effect should re-run:- Empty array (
[]
): Effect runs only once (on mount). - Array with dependencies: Effect runs when dependencies change.
- No array: Effect runs after every render (not recommended unless necessary).
- Empty array (
Common Use Cases:
1. Fetching Data:
import React, { useState, useEffect } from 'react'; function DataFetcher() { const [data, setData] = useState([]); useEffect(() => { fetch('https://jsonplaceholder.typicode.com/posts') .then((response) => response.json()) .then((data) => setData(data)); }, []); // Empty array ensures the effect runs only on mount return ( <ul> {data.map((item) => ( <li key={item.id}>{item.title}</li> ))} </ul> ); } export default DataFetcher;
2. Subscribing to Events:
import React, { useState, useEffect } from 'react'; function WindowSize() { const [width, setWidth] = useState(window.innerWidth); useEffect(() => { const handleResize = () => setWidth(window.innerWidth); window.addEventListener('resize', handleResize); // Cleanup function to remove the event listener return () => { window.removeEventListener('resize', handleResize); }; }, []); // Empty array ensures this effect is added only once return <p>Window width: {width}px</p>; } export default WindowSize;
3. Cleanup Effects:
import React, { useState, useEffect } from 'react'; function Timer() { const [count, setCount] = useState(0); useEffect(() => { const interval = setInterval(() => { setCount((prev) => prev + 1); }, 1000); // Cleanup function to stop the interval return () => clearInterval(interval); }, []); // Empty array ensures the effect is set up only once return <p>Seconds elapsed: {count}</p>; } export default Timer;
Key Points:
- Side Effects: Ideal for tasks that don’t directly affect rendering, like data fetching, setting up subscriptions, or manually modifying the DOM.
- Cleanup Function: Always include cleanup logic if the effect involves subscriptions or asynchronous operations to prevent memory leaks.
- Dependency Array: Properly specify dependencies to avoid unnecessary re-renders or stale values.