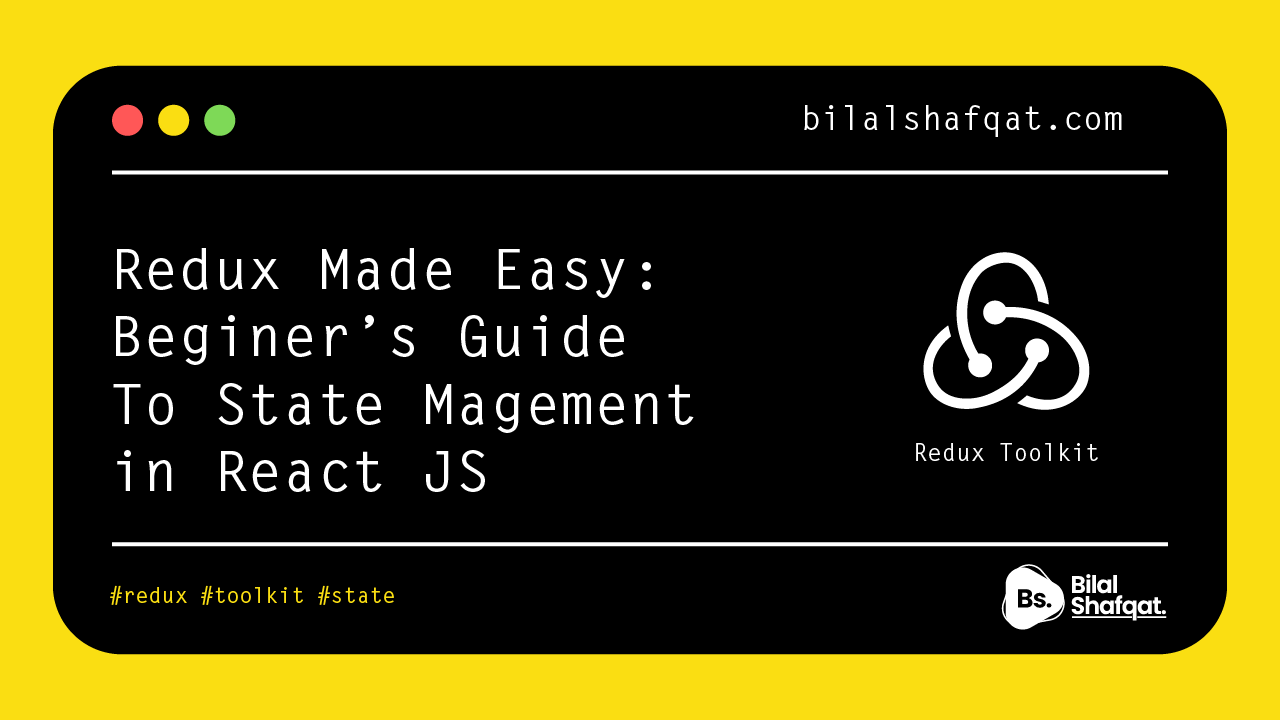
Redux Made Easy: Beginner’s Guide to State Management in React JS
- bilalshafqat42
- November 9, 2023
- React Js, Redux
- 1 Comment
Table of Contents
Redux made easy: Beginer's guide to state management in React JS
Redux is a powerful state management library often used in React applications. To help you get started with Redux, we’ll walk through the process of creating a simple counter application. By the end of this guide, you’ll have a solid understanding of Redux concepts like actions, reducers, and the store.
Step 1: Setting Up the Project
Before we dive into Redux, let’s set up a basic React project.
1. Initialize a New React Project:
npx create-react-app redux-counter
cd redux-counter
npm install redux react-redux
Step 2: Understanding Redux Concepts
- Store: The store holds the entire state of the application.
- Actions: Actions are plain JavaScript objects that describe a type and any payload needed to change the state
- Reducers: Reducers are pure functions that take the current state and an action and return a new state.
Step 3: Creating the Redux Store
mkdir src/redux
src/redux
folder, create a file called store.js:
import { createStore } from 'redux';
// Initial state
const initialState = {
count: 0,
};
// Reducer function
function counterReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
// Create Redux store
const store = createStore(counterReducer);
export default store;
count
of 0. The counterReducer
function handles two actions: INCREMENT
and DECREMENT
, which update the count
accordingly. Step 4: Connecting Redux to React
Next, integrate Redux with your React app. Open src/index.js
and wrap the root component with the Provider
component from react-redux
:
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './redux/store';
ReactDOM.render(
,
document.getElementById('root')
);
Step 5: Creating the Counter Component
Now, let’s create the counter component. Open src/App.js
and add the following code:
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
function App() {
// Access state and dispatch from Redux
const count = useSelector((state) => state.count);
const dispatch = useDispatch();
return (
Redux Counter
{count}
);
}
export default App;
In this component:
useSelector
is used to select thecount
from the Redux state.useDispatch
is used to dispatch actions to the Redux store.
Step 6: Running the Application
npm start
Conclusion
By building this simple counter application, you’ve covered the essential concepts of Redux, including actions, reducers, and the store. Redux may seem complex at first, but with practice, it becomes an invaluable tool for managing state in larger applications. Happy coding!
Related Posts
Most Useful React Hooks
- bilalshafqat42
- January 27, 2025
Most Useful React Hooks Discover the most essential React Hooks that every developer should kno ..
Structuring Your React App Like a Pro
- bilalshafqat42
- February 18, 2025
Introduction: Why App Structure Matters When developing a React application, maintaining a well ..
A WordPress Commenter
Hi, this is a comment.
To get started with moderating, editing, and deleting comments, please visit the Comments screen in the dashboard.
Commenter avatars come from Gravatar.