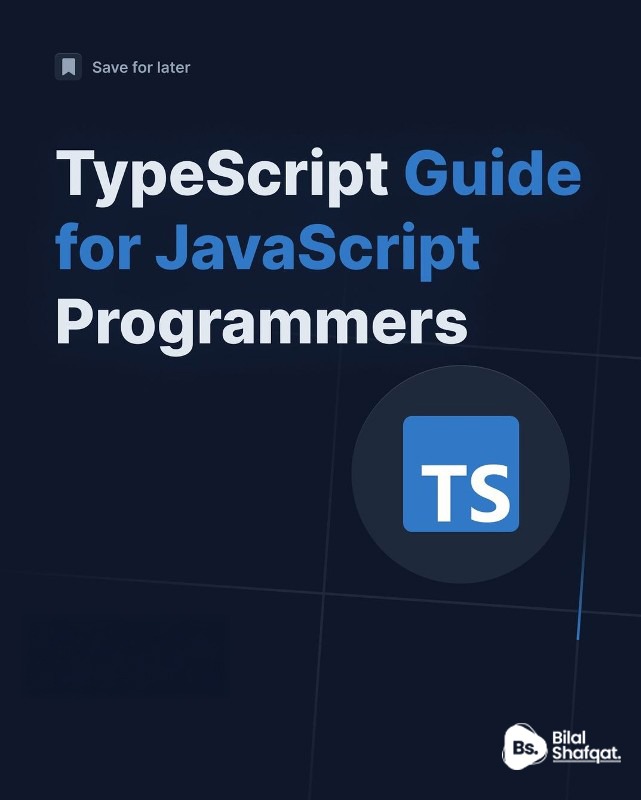
TypeScript Guide for JavaScript Programmers 🚀
- bilalshafqat42
- January 14, 2025
- Typescript
- 0 Comments
Introduction
JavaScript is a powerful language for web development, but as projects grow, managing complex codebases can become challenging. Enter TypeScript, a superset of JavaScript designed to add static typing, improve readability, and reduce bugs. This guide provides a comprehensive introduction to TypeScript for JavaScript developers, covering essential concepts like type inference, type definitions, unions, generics, and structural typing.
What is TypeScript?
TypeScript is an open-source programming language developed by Microsoft. It builds on JavaScript by adding optional static typing, making it easier to catch errors during development rather than runtime. TypeScript code compiles to plain JavaScript, ensuring compatibility with any environment that supports JavaScript.
Key Benefits of TypeScript:
- Type Safety: Helps prevent runtime errors by catching issues during compilation.
- Better Tooling: Enhanced IntelliSense and autocompletion in IDEs.
- Maintainability: Makes large codebases easier to manage and scale.
- Backward Compatibility: Works seamlessly with existing JavaScript libraries and frameworks.
Type Inference
TypeScript features type inference, which means it can automatically determine the type of a variable based on its initial value. This reduces the need for explicit type annotations, making code cleaner and more concise.
let calories = 89; // TypeScript infers 'number' let fruits = ["Banana", "Orange"]; // TypeScript infers 'string[]'
While inference simplifies code, you can still explicitly define types when needed, especially for function parameters or complex data structures.
Defining Types
TypeScript allows you to define types for variables, function parameters, and return values, improving code clarity.
interface Fruit { id: number; name: string; description?: string; // Marked optional with '?' } let fruit: Fruit = { id: 1, name: "Banana", description: "A healthy snack.", };
Defining types ensures that the data passed to and returned from functions is predictable and consistent.
Unions
Union types allow a variable to hold more than one type of value. This is useful when dealing with data that can vary between a few specific types.
type FruitSize = "S" | "M" | "L" let validSize: FruitSize = "M" let invalidSize: FruitSize = "Medium"; // Syntax error: "Medium" is not assignable to type 'FruitSize'.
Unions provide flexibility while maintaining type safety.
Generics
Generics let you create reusable components that work with multiple types while maintaining type safety. They are particularly useful for creating type-safe data structures like arrays or custom utilities.
interface Fruit { id: number; name: string; } interface Vegetable { id: number; color: string; } interface Page<T> { data: T[]; } let fruit1: Fruit = { id: 1, name: "Banana" }; let fruit2: Fruit = { id: 2, name: "Orange" }; let vegetable1: Vegetable = { id: 1, color: "Green" }; let vegetable2: Vegetable = { id: 2, color: "Red" }; let fruitsPage: Page<Fruit> = { data: [fruit1, fruit2], }; let vegetablesPage: Page<Vegetable> = { data: [vegetable1, vegetable2], };
Here, the identity
function works with any type (T
), and the type is determined when the function is called.
Structural Types
TypeScript uses a structural typing system, meaning compatibility between types is determined by their structure rather than their explicit declarations.
For example, if two objects have the same shape, they are considered compatible.
function eatFruit(fruit: Fruit){ console.log( `Yummy! ${fruit.name} is delicious` ) } eatFruit({ id: 1, name: "Banana" });
This flexibility makes TypeScript highly intuitive and adaptable for real-world scenarios.
Conclusion
TypeScript is a game-changer for JavaScript developers, offering powerful tools to enhance code quality and maintainability. By understanding its key features like type inference, type definitions, unions, generics, and structural typing, you can write cleaner, more reliable code.