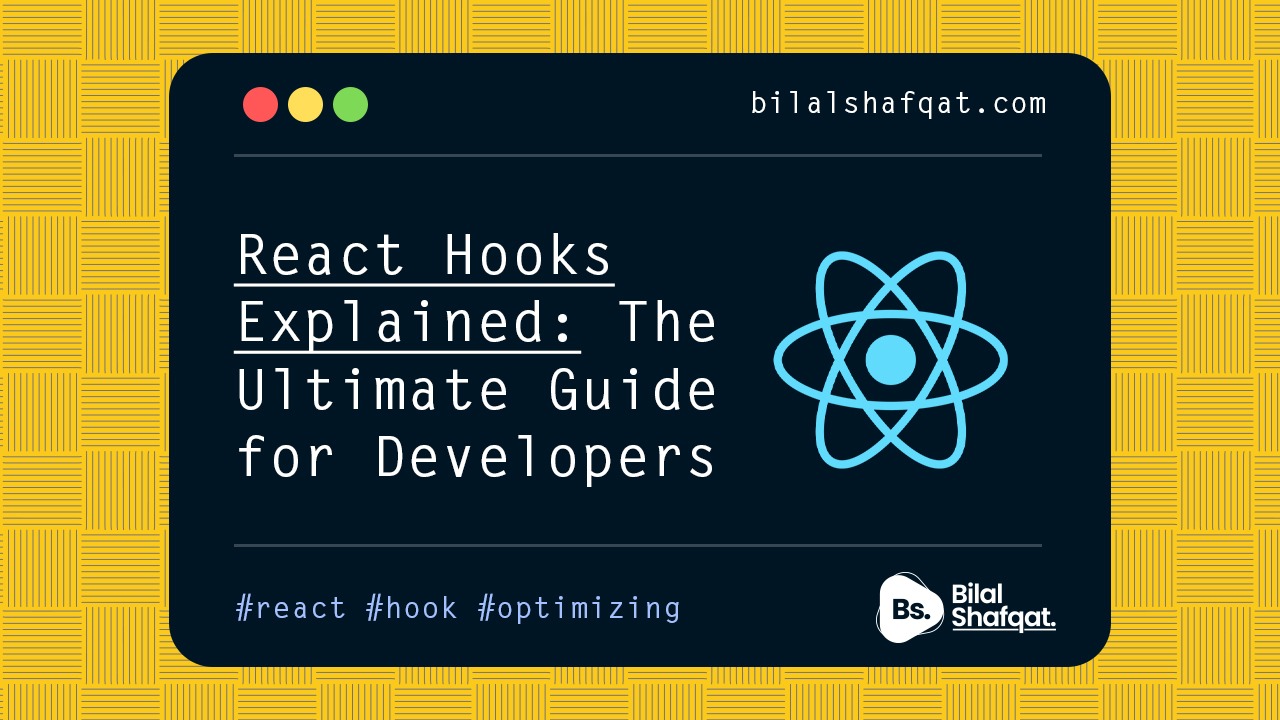
React Hooks Explained: The Ultimate Guide for Developers
- bilalshafqat42
- March 9, 2025
- React Js
- 0 Comments
Introduction
React Hooks revolutionized the way developers build React applications by enabling functional components to handle state and side effects without relying on class components. Since their introduction in React 16.8, Hooks have become a crucial part of modern React development.
In this article, we’ll explore React Hooks in depth, covering their purpose, how they work, best practices, and common mistakes to avoid, ensuring you can effectively integrate them into your projects.
What are React Hooks?
React Hooks are special JavaScript functions that allow functional components to use state, lifecycle methods, and other React features without needing a class component. They make React code cleaner, more reusable, and easier to manage.
Why Use React Hooks?
Hooks bring several advantages to React development, including:
- Simplified Code: No need for complex class components.
- Better Performance: Optimized re-rendering and memoization.
- Reusable Logic: Custom Hooks enable reusable stateful logic.
- Cleaner Component Structure: Improved readability and maintainability.
- Easier Testing: Functional components with Hooks are easier to test than class components.
Key React Hooks Explained and Their Usage
1. useState – Managing Local State
The useState
Hook allows functional components to store and update state.
import { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase</button> </div> ); }
2. useEffect – Handling Side Effects
The useEffect
Hook is used to handle side effects like data fetching, updating the DOM, or subscribing to events.
import { useEffect } from 'react'; function Example() { useEffect(() => { console.log("Component Mounted"); }, []); // Runs once when the component mounts return <div>Hello, World!</div>; }
Best Practices for useEffect:
- Use dependency arrays to control execution.
- Cleanup subscriptions to avoid memory leaks.
- Separate concerns by using multiple
useEffect
calls.
3. useContext – Avoiding Props Drilling
useContext
simplifies state sharing between components without passing props manually.
import { useContext } from 'react'; import { ThemeContext } from './ThemeContext'; function ThemedComponent() { const theme = useContext(ThemeContext); return <div style={{ backgroundColor: theme.background }}>Themed Component</div>; }
4. useRef – Accessing DOM Elements & Persisting Values
The useRef
Hook allows direct interaction with DOM elements without causing re-renders.
import { useRef } from 'react'; function FocusInput() { const inputRef = useRef(null); const focusInput = () => { inputRef.current.focus(); }; return ( <div> <input ref={inputRef} type="text" /> <button onClick={focusInput}>Focus Input</button> </div> ); }
5. useReducer – Managing Complex State Logic
The useReducer
Hook is an alternative to useState
for handling complex state logic.
import { useReducer } from 'react'; const initialState = { count: 0 }; function reducer(state, action) { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; default: return state; } } function Counter() { const [state, dispatch] = useReducer(reducer, initialState); return ( <div> <p>Count: {state.count}</p> <button onClick={() => dispatch({ type: 'increment' })}>+</button> <button onClick={() => dispatch({ type: 'decrement' })}>-</button> </div> ); }
6. useMemo – Optimizing Performance
The useMemo
Hook memoizes expensive calculations to improve performance.
import { useMemo } from 'react'; function ExpensiveCalculation({ num }) { const squaredValue = useMemo(() => { console.log("Calculating..."); return num * num; }, [num]); return <p>Squared Value: {squaredValue}</p>; }
7. useCallback – Memoizing Functions
The useCallback
Hook prevents unnecessary function re-creations.
import { useCallback } from 'react'; function ParentComponent() { const handleClick = useCallback(() => { console.log("Button clicked!"); }, []); return <button onClick={handleClick}>Click Me</button>; }
Common Mistakes to Avoid with React Hooks
- Forgetting Dependency Arrays in useEffect: Leads to infinite loops or unnecessary re-executions.
- Mutating State Directly: Always use the state setter function.
- Not Using Memoization for Performance Optimization: Leads to redundant computations.
- Using Hooks in Loops or Conditions: Always call Hooks at the top level of the component.
Conclusion
React Hooks simplify the development process by eliminating class components’ complexity while making functional components more powerful. By mastering Hooks, developers can build more maintainable and scalable applications efficiently.
FAQs
1. What are the benefits of using React Hooks?
- Simplifies code by eliminating class components
- Improves readability and reusability
- Enhances performance with
useMemo
anduseCallback
- Makes component logic easier to share via custom Hooks
2. Can I use Hooks inside class components?
No, Hooks are exclusive to functional components.
3. What is the difference between useEffect and useLayoutEffect?
useEffect
runs asynchronously after render, while useLayoutEffect
runs synchronously before the browser paints the screen.
4. How can I share state logic between components?
You can use the Context API with useContext
or create custom Hooks.