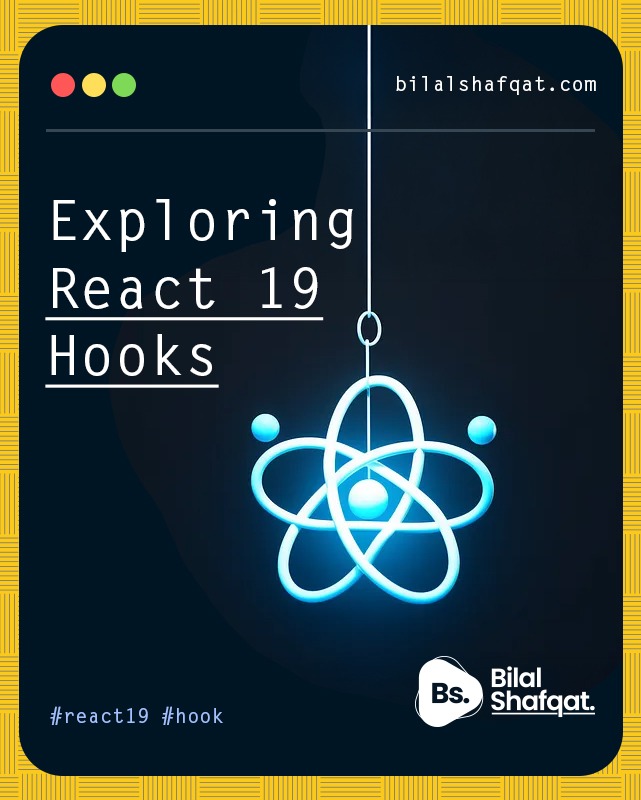
Exploring React 19 Hooks
- bilalshafqat42
- January 16, 2025
- React Js
- 0 Comments
React 19 introduced some exciting hooks that streamline state management and interactivity for modern web applications. In this article, we’ll explore useFormStatus
, useActionState
, useOptimistic
, and use
. Each of these hooks enhances user experience and developer productivity. Let’s dive into their usage with examples.
1. useFormStatus
The useFormStatus
hook simplifies the process of tracking the submission status of a form. It allows you to provide feedback to users while forms are being processed.
import { useFormStatus } from "react"; // Import the useFormStatus hook from React. function MyFormComponent() { // Destructure the properties from useFormStatus hook. // - `pending`: Tracks if the form is in the process of being submitted. // - `error`: Contains any error that occurred during submission. // - `success`: Indicates if the form was submitted successfully. const { pending, error, success } = useFormStatus(); return ( <form> {/* If form is pending submission, display a message */} {pending && <p>Submitting....</p>} {/* If an error occurred during submission, display the error message */} {error && <p>Error: {error.message}</p>} {/* If form submission is successful, display success message */} {success && <p>Form submitted successfully!</p>} </form> ); }
Code Explanation:
import { useFormStatus }
: This is importing theuseFormStatus
hook from React (assuming it’s part of future React features).const { pending, error, success } = useFormStatus();
: TheuseFormStatus
hook is used to track the state of the form submission, including whether it’s pending, has encountered an error, or was successful.{pending && <p>Submitting....</p>}
: If the form submission is in progress, a “Submitting…” message is displayed.{error && <p>Error: {error.message}</p>}
: If there was an error during the submission, an error message is shown with the details fromerror.message
.{success && <p>Form submitted successfully!</p>}
: If the form was submitted successfully, a success message is displayed.
Key Points:
pending
tells if the form submission is in progress.- You can use it to disable the submit button or show a loading state.
2. useActionState
useActionState
is helpful for tracking the state of an ongoing action, such as button clicks triggering async operations.
import { useActionState } from "react"; // Import the useActionState hook from React. function MyActionComponent() { // Destructure the properties from useActionState hook. // - `pending`: Indicates if the action is currently in progress. // - `error`: Contains any error that occurred during the action. // - `success`: Indicates if the action was completed successfully. // We pass a unique name (e.g., "myActionName") to track the specific action. const { pending, error, success } = useActionState("myActionName"); return ( <div> {/* If the action is in progress, display a "pending" message */} {pending && <p>Action in progress</p>} {/* If an error occurred during the action, display the error message */} {error && <p>Error: {error.message}</p>} {/* If the action was completed successfully, display a success message */} {success && <p>Action completed successfully</p>} </div> ); }
Code Explanation:
import { useActionState }
: This line imports theuseActionState
hook from React (or a library if it’s part of a third-party package). This hook is used to manage the state of an action, such as a network request or a state-changing operation.const { pending, error, success } = useActionState("myActionName");
:- The
useActionState
hook tracks the state of an action based on the name you provide ("myActionName"
). - The hook returns three properties:
pending
,error
, andsuccess
, which are used to display the state of the action.
- The
{pending && <p>Action in progress</p>}
: Displays a message if the action is currently in progress (e.g., an API request or background task).{error && <p>Error: {error.message}</p>}
: Displays an error message if there was an issue with the action, such as a network failure or invalid data.{success && <p>Action completed successfully</p>}
: Displays a success message when the action has been completed without errors.
Key Points:
useActionState
monitors a specific action by name (e.g.,'save-data'
).- Use it to manage UI feedback like disabling buttons or showing a spinner.
3. useOptimistic
useOptimistic
enables optimistic updates, allowing your UI to update immediately before waiting for server confirmation. It’s useful for enhancing user experience by minimizing delays.
import { useOptimistic } from "react"; // Import the useOptimistic hook from React. function MyOptimisticComponent() { // useOptimistic hook is used to optimistically update the state while the action is in progress. // - The first argument is the initial state ({ count: 0 }). // - The second argument is a function that describes how to update the state. // It takes two arguments: `currentState` (the previous state) and `newUpdate` (the change to apply). const [optimisticState, setOptimisticState] = useOptimistic( { count: 0 }, // Initial state: the count starts at 0 (currentState, newUpdate) => ({ count: currentState.count + newUpdate }) // State update function: increments count ); return ( <div> {/* Display the current count */} <p>Count: {optimisticState.count}</p> {/* When the button is clicked, optimistically update the state by incrementing the count */} <button onClick={() => setOptimisticState(1)}>Increment</button> </div> ); }
Code Explanation:
import { useOptimistic }
: This line imports theuseOptimistic
hook. This hook is used to optimistically update a piece of state, providing a temporary value until the action completes.const [optimisticState, setOptimisticState] = useOptimistic(...)
:- The
useOptimistic
hook returns an array containing the current state (optimisticState
) and a setter function (setOptimisticState
). - First argument:
{ count: 0 }
is the initial state, setting the count to 0 when the component first renders. - Second argument: A function that receives the
currentState
andnewUpdate
as arguments and returns the updated state. In this case, it increments thecount
by the value ofnewUpdate
(which is 1 when the button is clicked).
- The
<p>Count: {optimisticState.count}</p>
: Displays the currentcount
from theoptimisticState
. This value will immediately update in the UI whensetOptimisticState
is called.<button onClick={() => setOptimisticState(1)}>Increment</button>
: When the button is clicked, it callssetOptimisticState(1)
, which updates the state optimistically by incrementing thecount
by 1.
Key Points:
useOptimistic
takes the initial state and an updater function.- Updates are immediately reflected in the UI, enhancing perceived speed.
- Rollback logic can be added in case of server failure.
4. use
The use
hook simplifies handling promises in React components, especially for fetching data or suspense-based features.
import { use } from "react"; // Hypothetical future React feature async function fetchData() { const response = await fetch("/api/data"); return response.json(); } function MyDataComponent() { // Assuming `use` could be used to directly fetch data const data = use(fetchData()); // `use` would handle async fetching return ( <div> <p>Data: {JSON.stringify(data)}</p> </div> ); } export default MyDataComponent;
Key Points:
use
resolves promises directly within the component.- Useful in server components and with Suspense for async data fetching.
- Avoids the need for additional state or effects to manage loading states.
Conclusion
React 19 hooks like useFormStatus
, useActionState
, useOptimistic
, and use
bring new capabilities for managing state and enhancing user interactions. These hooks simplify handling form states, actions, optimistic updates, and async data fetching, making your components more intuitive and responsive.
Embrace these hooks in your projects to unlock a smoother developer experience and richer user interfaces.